Python is one of the most popular programming languages in the world, known for its simplicity and versatility. Whether you’re a complete beginner or an experienced developer, understanding Python can unlock a plethora of opportunities in technology, data science, web development, and more. This article aims to provide a comprehensive guide to Python, covering everything from its basics to advanced applications. Dive in and discover how to maximize your potential with this powerful language.
Table of Contents
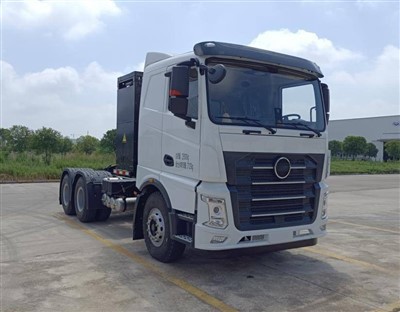
- What is Python?
- History of Python
- Features of Python
- Installation and Setup
- Python Syntax
- Data Types and Variables
- Control Structures
- Functions
- Modules and Packages
- Real-World Applications of Python
- FAQ
What is Python?
Python is a high-level, interpreted programming language known for its ease of learning and readability. It is designed to be simple and straightforward, making it an excellent choice for first-time programmers while remaining powerful enough for experienced developers to create complex applications.
History of Python
Python was created by Guido van Rossum and first released in 1991. Since then, it has evolved dramatically, becoming one of the leading programming languages by 2023. Understanding the history of Python can provide insights into its design philosophy and ongoing development.
The Birth of Python
The name ‘Python’ comes from the British comedy series “Monty Python’s Flying Circus,” which Guido enjoyed. The language was designed to be a successor to the ABC programming language, focusing on code readability and simplicity.
Key Milestones
Year | Event |
---|---|
1991 | Python 1.0 released |
2000 | Python 2.0 released, introducing garbage collection |
2008 | Python 3.0 released, aimed at fixing inconsistencies |
2020 | End of life for Python 2.x announced |
Features of Python
Python’s features make it uniquely suited for various applications:
- Easy to Learn: Python’s syntax is clear and intuitive.
- Interpreted Language: Python executes code line by line, which makes debugging easier.
- Extensive Libraries: Python offers a large standard library for various tasks, from web development to data analysis.
- Cross-Platform: Python runs on multiple operating systems, including Windows, macOS, and Linux.
- Object-Oriented: Python supports object-oriented programming, which encourages code reusability.
Installation and Setup
Getting started with Python is a straightforward process. Here’s how you can install it on different operating systems:
Windows
- Download the latest version of Python from the official website (python.org).
- Run the installer and ensure that you check the box that says “Add Python to PATH.”
- Open Command Prompt and type
python --version
to confirm the installation.
macOS
- Python 2.x comes pre-installed on macOS. To install Python 3.x, use Homebrew. First, install Homebrew by running a command in Terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Then, install Python 3 by typing:
brew install python
- Confirm installation by typing
python3 --version
.
Linux
- Most Linux distributions come with Python pre-installed. To check this, open a terminal and type
python --version
. - If Python is not installed, you can install it using:
sudo apt-get install python3
for Ubuntu or
sudo yum install python3
for Fedora.
Python Syntax
Python syntax is known for being clean and easy to read. Here are some fundamental concepts:
Comments
Comments in Python start with a # sign and are used to explain code.
Variables
In Python, variables do not require explicit declaration and can hold data of any type:
name = "Heil Python"
age = 5
Indentation
Python uses indentation to define the structure of code blocks. This is crucial for defining loops, functions, and conditionals.
Data Types and Variables
Python supports several built-in data types:
Primitive Data Types
- Integers: Whole numbers (e.g.,
x = 10
) - Floats: Decimal numbers (e.g.,
y = 10.5
) - Strings: Text data (e.g.,
name = "Hello"
) - Booleans: Represents True or False values.
Complex Data Types
- Lists: Ordered collections (e.g.,
my_list = [1, 2, 3]
) - Tuples: Immutable ordered collections (e.g.,
my_tuple = (1, 2, 3)
) - Dictionaries: Key-value pairs (e.g.,
my_dict = {'name': 'Alice', 'age': 25}
) - Sets: Unordered collections of unique elements (e.g.,
my_set = {1, 2, 3}
)
Control Structures
Control structures are essential for making decisions in your code.
Conditional Statements
Use conditional statements to execute code based on certain conditions:
if age > 18: print("Adult") else: print("Minor")
Loops
Python provides for
and while
loops to iterate over sequences:
for i in range(5): print(i)
Functions
Functions allow you to encapsulate code for reuse. You can define a function using the def
keyword:
def greet(name): return "Hello " + name
Lambda Functions
Python supports anonymous functions known as lambda functions:
square = lambda x: x ** 2 print(square(5))
Modules and Packages
Modules allow you to organize code effectively. A package is a collection of modules.
Importing Modules
You can import modules using the import
statement:
import math print(math.sqrt(25))
Creating Your Own Module
To create a module, simply save your functions in a .py file and import them into your main application:
# my_module.py def add(x, y): return x + y
Real-World Applications of Python
Python is utilized in various fields:
Web Development
Frameworks like Django and Flask make web development seamless in Python.
Data Science
Libraries like Pandas, NumPy, and Matplotlib are widely used for data manipulation and visualization.
Machine Learning
With libraries such as TensorFlow and Scikit-learn, Python is a go-to language for machine learning projects.
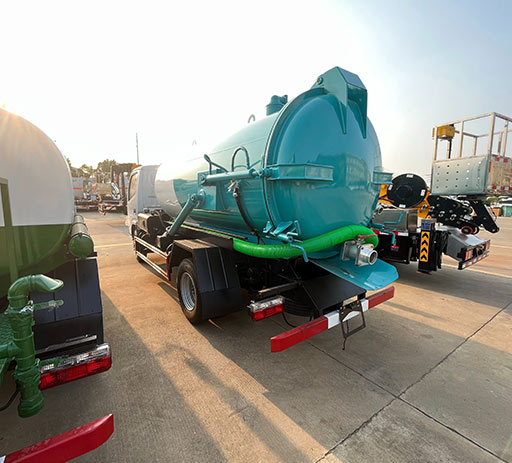
Automation/Scripting
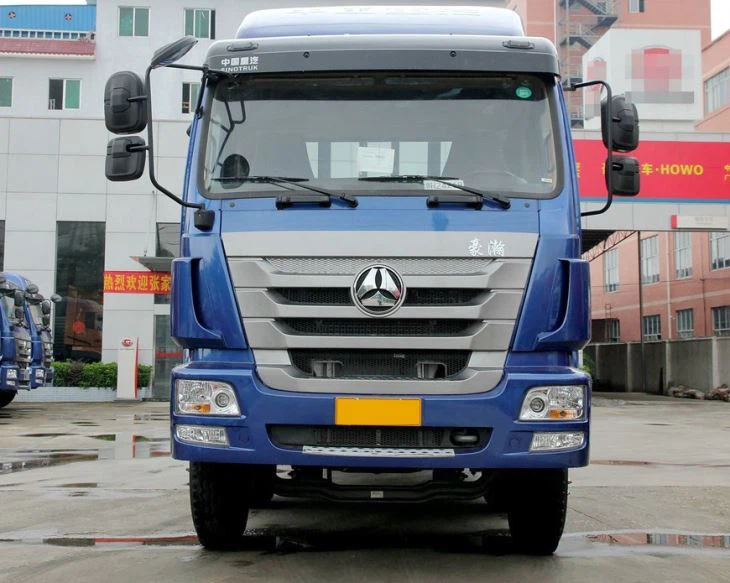
Python excels in writing scripts to automate boring tasks, enhancing productivity.
Game Development
Libraries like Pygame make Python a suitable choice for game development.
FAQ
1. What are the benefits of using Python over other programming languages?
Python is user-friendly, has extensive libraries, and supports various programming paradigms, making it versatile for different projects.
2. Is Python suitable for beginners?
Yes, Python’s straightforward syntax and community resources make it an excellent choice for beginners in programming.
3. Can Python be used for mobile app development?
While Python is not the primary language for mobile app development, tools like Kivy and BeeWare allow you to build mobile applications.
4. What are some popular libraries I should know?
Some popular libraries include NumPy for numerical computing, Pandas for data manipulation, Matplotlib for plotting, and TensorFlow for machine learning.
5. How can I improve my Python skills?
Practice coding regularly, work on projects, read documentation, and participate in online coding communities or workshops.
6. Is Python a good choice for web development?
Absolutely! Python offers powerful frameworks such as Django and Flask that facilitate web development.